Translate, rotate image
Peponi │ 2/25/2025 │ 4m
C#
NugetPackageOpenCvSharp4
Translate, rotate image
2/25/2025
4m
Peponi
C#
NugetPackageOpenCvSharp4
1. Introduction
OpenCvSharp4는 변환 함수를 제공하여 이미지 이동, 회전 등을 할 수 있다. 이 문서에서는 Cv2.WrapAffine()
, Cv2.Rotate()
메서드를 통해 이미지를 이동하고 회전하는 방법을 간략하게 알아본다.
실습에 사용할 이미지는 다음과 같다.
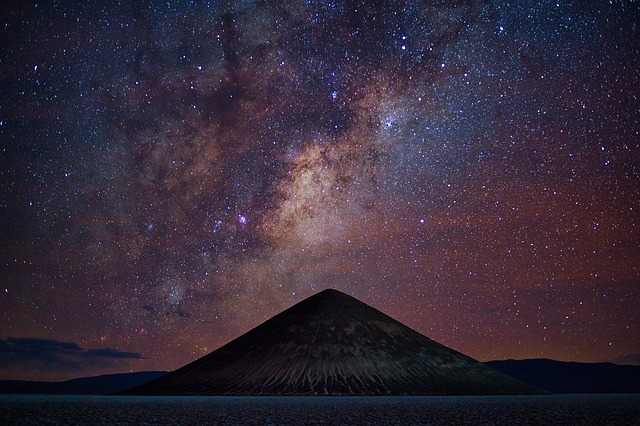
2. Translation
Translation을 수행하여 이미지의 X, Y축 위치를 변경할 수 있다. 아핀 변환을 통해 수행하며, 다음 연산을 수행한다.
private void Translate(Mat image)
{
// 단위행렬 생성 (1행 : [1, 0, 0], 2행 : [0, 1, 0])
using Mat translation = Mat.Eye(2, 3, MatType.CV_32F);
// Set(row, col, value)
translation.Set<float>(0, 2, 150); // 1행 : [1, 0, 150]
translation.Set<float>(1, 2, 50); // 2행 : [0, 1, 50]
// Translate
using var result = new Mat();
Cv2.WarpAffine(image, result, translation, new OpenCvSharp.Size(image.Width, image.Height));
}
3. Rotation
이미지를 단순히 90 단위로 회전하려는 경우 OpenCvSharp4에 내장된 Cv2.Rotate()
메서드를 이용할 수 있다.
private void Rotate(Mat image)
{
using var result = new Mat();
Cv2.Rotate(image, result, RotateFlags.Rotate90Clockwise);
}
RotateFlags
는 다음 표를 참조한다.
RotateFlags | Value | Description |
---|---|---|
Rotate90Clockwise | 0 | Rotate 90 degrees clockwise |
Rotate180 | 1 | Rotate 180 degrees clockwise |
Rotate90Counterclockwise | 2 | Rotate 270 degrees clockwise |
4. Rotation with affine transformation
이미지를 특정 각도로 회전하려는 경우 OpenCvSharp4에서 제공하는 Cv2.GetRotationMatrix2D()
를 통해 변환 행렬을 생성하여 아핀 변환을 수행할 수 있다. 이 때 수행되는 연산은 기본적으로 다음과 같다.
Cv2.GetRotationMatrix2D()
에는 회전 중심과 스케일 기능이 포함되어 있어 최종적으로 수행되는 연산은 다음과 같다.
private void RotateWithAngle(Mat image)
{
// GetRotationMatrix2D(center, angle: CCW, scale)
using var rotation = Cv2.GetRotationMatrix2D(new Point2f(image.Width / 2, image.Height / 2), 60, 1);
// Rotate
using var result = new Mat();
Cv2.WarpAffine(image, result, rotation, new OpenCvSharp.Size(image.Width, image.Height));
}