Contour detection
Peponi │ 5/21/2025 │ 4m
Contour detection
Peponi
1. Introduction
Contour는 이미지 내에서 비슷한 픽셀 값을 가지는 영역의 경계선을 의미한다. 즉, edge가 연결되어 하나의 도형을 이루는 형태로 Contour detection
은 이 영역을 찾아내는 기술을 뜻한다. Contour detection을 통해 이미지 상 객체의 전반적인 형태, 구조를 이해할 수 있으며 의료, 공장 자동화, 자율 주행 등에 활용할 수 있다.
Contour detection을 수행할 때는 binary 이미지를 활용한다. 간단하게 contour를 검출할 수 있는 경우에는 일반적인 thresholding 과정을 거친 이미지를 사용하기도 하지만, 경우에 따라 edge detection 이미지를 input 이미지로 활용하여 검출 정확도를 높이기도 한다.
이 문서에서는 두 가지 경우에 대한 예시와 함께 윤곽선 탐색 및 근사 방법 설정에 대해 알아본다.
실습에 사용할 이미지는 다음과 같다.
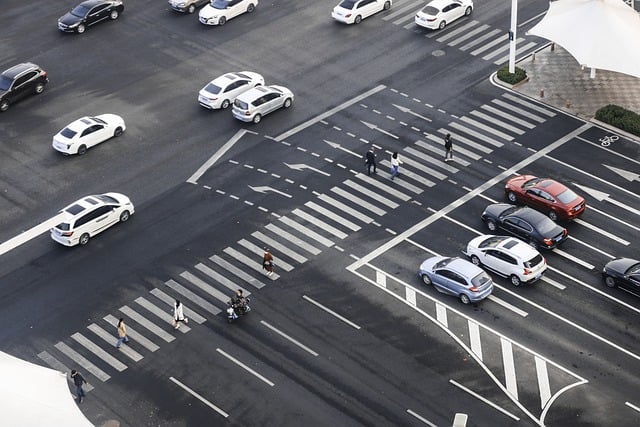
2. Binary image
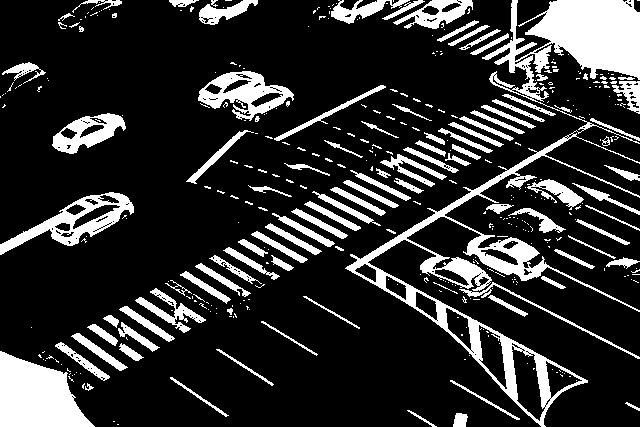
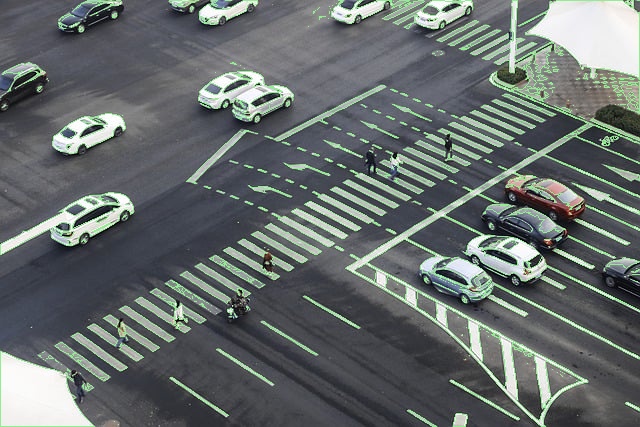
Contour detection을 수행하기 위한 전처리는 주로 adaptive thresholding이 사용되며, 이미지의 특성에 따라 적절한 thresholding 처리가 필요하다. 이 섹션에서는 Otsu의 이진화 방법을 적용한 이미지를 이용하여 contour detection을 수행하는 예시를 보여준다.
private void FindContours(Mat image)
{
using var grayscale = image.CvtColor(ColorConversionCodes.BGR2GRAY);
using var threshold = grayscale.Threshold(-1, 255, ThresholdTypes.Binary | ThresholdTypes.Otsu);
// Contour detection
threshold.FindContours(out var contours, out var hierarchy, RetrievalModes.List, ContourApproximationModes.ApproxTC89KCOS);
using var contoursImage = image.Clone();
contoursImage.DrawContours(contours, -1, Scalar.LightGreen);
Cv2.ImShow("Threshold", threshold);
Cv2.ImShow("Contours", contoursImage);
}
Mat.FindContours()
를 호출할 때 사용하는 RetrievalModes
, ContourApproximationModes
에 대해서는 다음 표를 참조한다.
RetrievalModes | Details |
---|---|
External | Retrieves only the extreme outer contours. It sets for all the contours |
List | Retrieves all of the contours without establishing any hierarchical relationships |
CComp | Retrieves all of the contours and organizes them into a two-level hierarchy. At the top level, there are external boundaries of the components. At the second level, there are boundaries of the holes. If there is another contour inside a hole of a connected component, it is still put at the top level |
Tree | Retrieves all of the contours and reconstructs a full hierarchy of nested contours |
FloodFill |
ContourApproximationModes | Details |
---|---|
ApproxNone | Translate all the points from the chain code into points |
ApproxSimple | Compress horizontal, vertical, and diagonal segments, that is, the function leaves only their ending points |
ApproxTC89L1 | Apply one of the flavors of Teh-Chin chain approximation algorithm |
ApproxTC89KCOS | Apply one of the flavors of Teh-Chin chain approximation algorithm |
3. Edge image
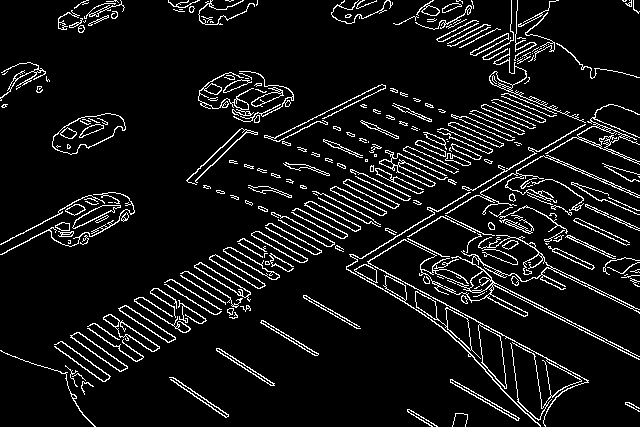
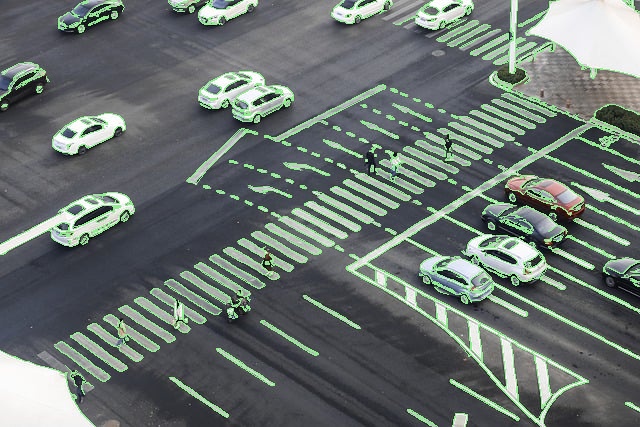
앞선 thresholding 방법에서는 차량의 색상에 따라 검출 정확도가 달라지는 경향이 있었다. 이 섹션에서는 contour detection의 정확도를 높이기 위해 edge detection 이미지를 이용하여 검출을 수행하는 예시를 보여준다.
private void FindContoursWithCanny(Mat image)
{
using var grayscale = image.CvtColor(ColorConversionCodes.BGR2GRAY);
using var canny = grayscale.Canny(250, 500);
// Contour detection
canny.FindContours(out var contours, out var hierarchy, RetrievalModes.List, ContourApproximationModes.ApproxTC89KCOS);
using var contoursImage = image.Clone();
contoursImage.DrawContours(contours, -1, Scalar.LightGreen);
Cv2.ImShow("Canny", canny);
Cv2.ImShow("Contours with Canny", contoursImage);
}